创建项目框架
1
2
3
4
5
6
7
8
|
# pnpm 本次使用包管理工具,后续都采用 pnpm
pnpm create vie
# 或者 yarn
yarn create vite
# 或者 npm
npm creat vite
|
1
2
3
4
|
# 安装依赖
pnpm install
# 启动
pnpm run dev
|
设置路径别名
src/vite.config.js
1
2
3
4
5
6
7
8
9
10
11
12
13
|
import { defineConfig } from 'vite'
import vue from '@vitejs/plugin-vue'
// https://vite.dev/config/
export default defineConfig({
plugins: [vue()],
// 设置路径别名
resolve: {
alias: {
'@': '/src',
}
}
})
|
为了能实现鼠标点击路径跳转,需要安装 别名路径跳转
设置路由
删除 App.vue
多余内容,HelloWorld.vue
,添加 vue-router
依赖
新建一个组件
src/views/Main.vue
1
2
3
4
5
6
7
8
9
10
11
12
13
|
<template>
<div>
<h1>这是Main组件</h1>
</div>
</template>
<script setup>
</script>
<style scoped>
</style>
|
设置路由
src/router/index.js
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
|
import { createRouter, createWebHashHistory } from "vue-router"
// 路由规则
const routes = [
{
// main 组件
path: '/',
name: 'main',
component: () => import("@/views/Main.vue")
}
]
// 导出路由配置
const router = createRouter({
// 设置路由模式
history: createWebHashHistory(),
// 设置路由,同名,后面不用写
routes,
})
export default router;
|
引入路由
src/main.js
1
2
3
4
5
6
|
import { createApp } from 'vue'
import App from './App.vue'
import router from './router'
const app = createApp(App)
app.use(router).mount('#app')
|
设置 App.vue
src/App.vue
1
2
3
4
5
6
7
8
9
10
|
<script setup>
</script>
<template>
<router-view></router-view>
</template>
<style >
</style>
|
访问 localhost:5173
查看是否成功
引入 element-plus
安装
1
|
pnpm install element-plus
|
按需导入之自动导入
安装unplugin-vue-components
和 unplugin-auto-import
这两款插件
1
|
pnpm install -D unplugin-vue-components unplugin-auto-import
|
src/vite.config.js
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
|
import { defineConfig } from 'vite'
import vue from '@vitejs/plugin-vue'
import AutoImport from 'unplugin-auto-import/vite'
import Components from 'unplugin-vue-components/vite'
import { ElementPlusResolver } from 'unplugin-vue-components/resolvers'
// https://vite.dev/config/
export default defineConfig({
plugins: [
vue(),
AutoImport({
resolvers: [ElementPlusResolver()],
}),
Components({
resolvers: [ElementPlusResolver()],
}),
],
// 设置路径别名
resolve: {
alias: {
'@': '/src',
}
}
})
|
主页添加按钮查看是否成功
src/views/Main.vue
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
|
<template>
<div>
<h1>这是Main组件</h1>
<div class="mb-4">
<el-button>Default</el-button>
<el-button type="primary">Primary</el-button>
<el-button type="success">Success</el-button>
<el-button type="info">Info</el-button>
<el-button type="warning">Warning</el-button>
<el-button type="danger">Danger</el-button>
</div>
</div>
</template>
<script setup>
</script>
<style scoped></style>
|
效果展示:
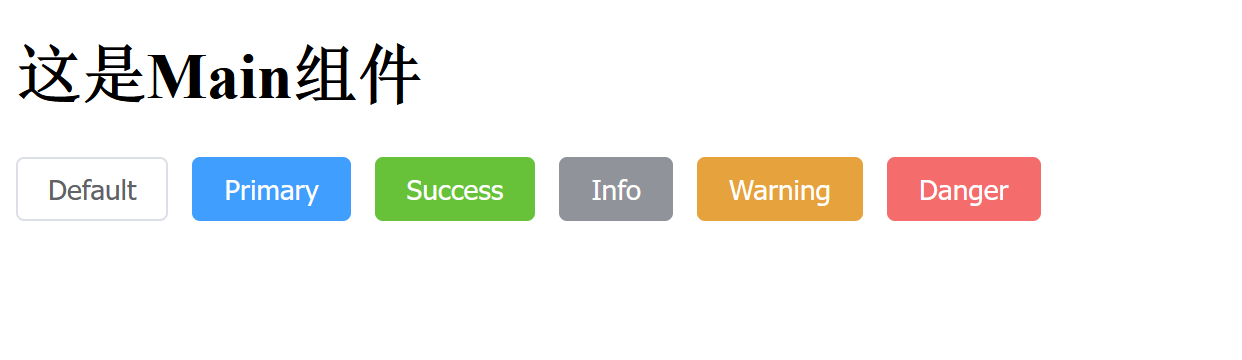